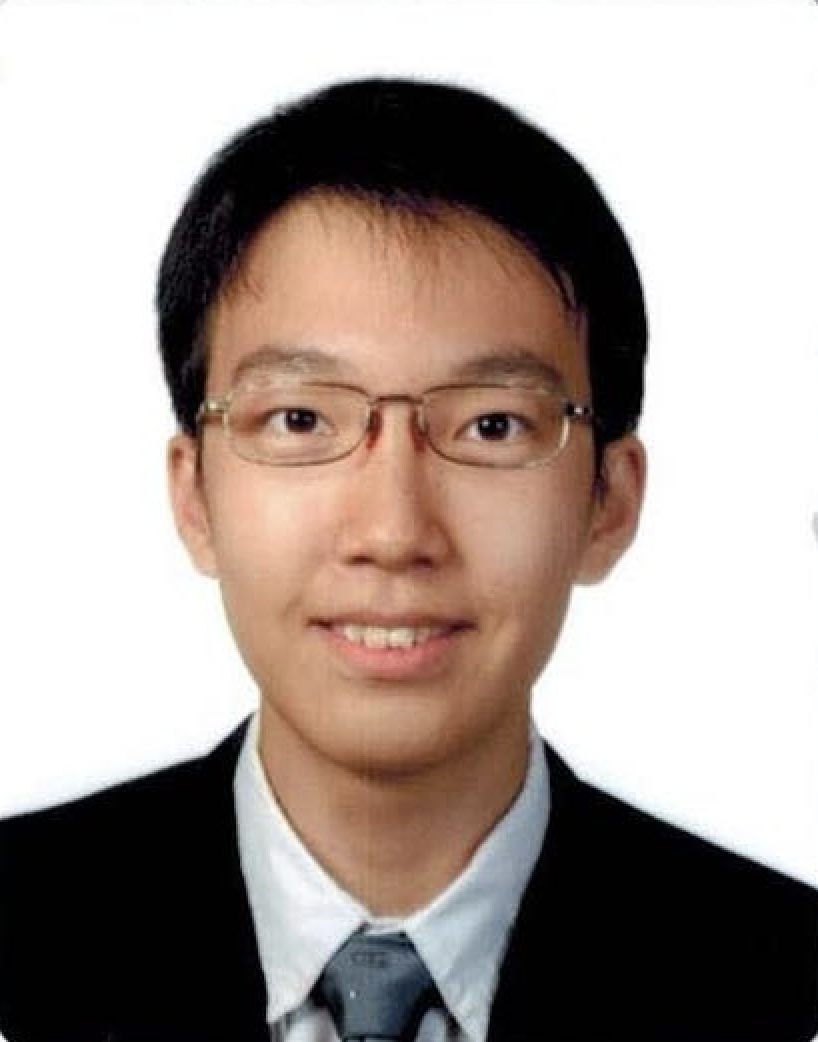
This page serves as my CS2103T project portfolio page on project Typee. The following technologies and software were used in the development of Typee application:
Overview
Typee is a desktop engagement management application. It has a GUI created with JavaFX, but most of the user interactions happen using a CLI (Command Line Interface).
It is an appointment manager for secretaries and receptionists to better schedule and manage appointments. Typee comes with a dynamic parsing input structure and productivity enhancing features like graphical calendar view,
conflict checker, sorting and undo/redo. All data required is stored locally, and Internet connection is not necessary for full functionality.
Typee is written in Java and contains around 17kLoC.
This is what our project looks like:
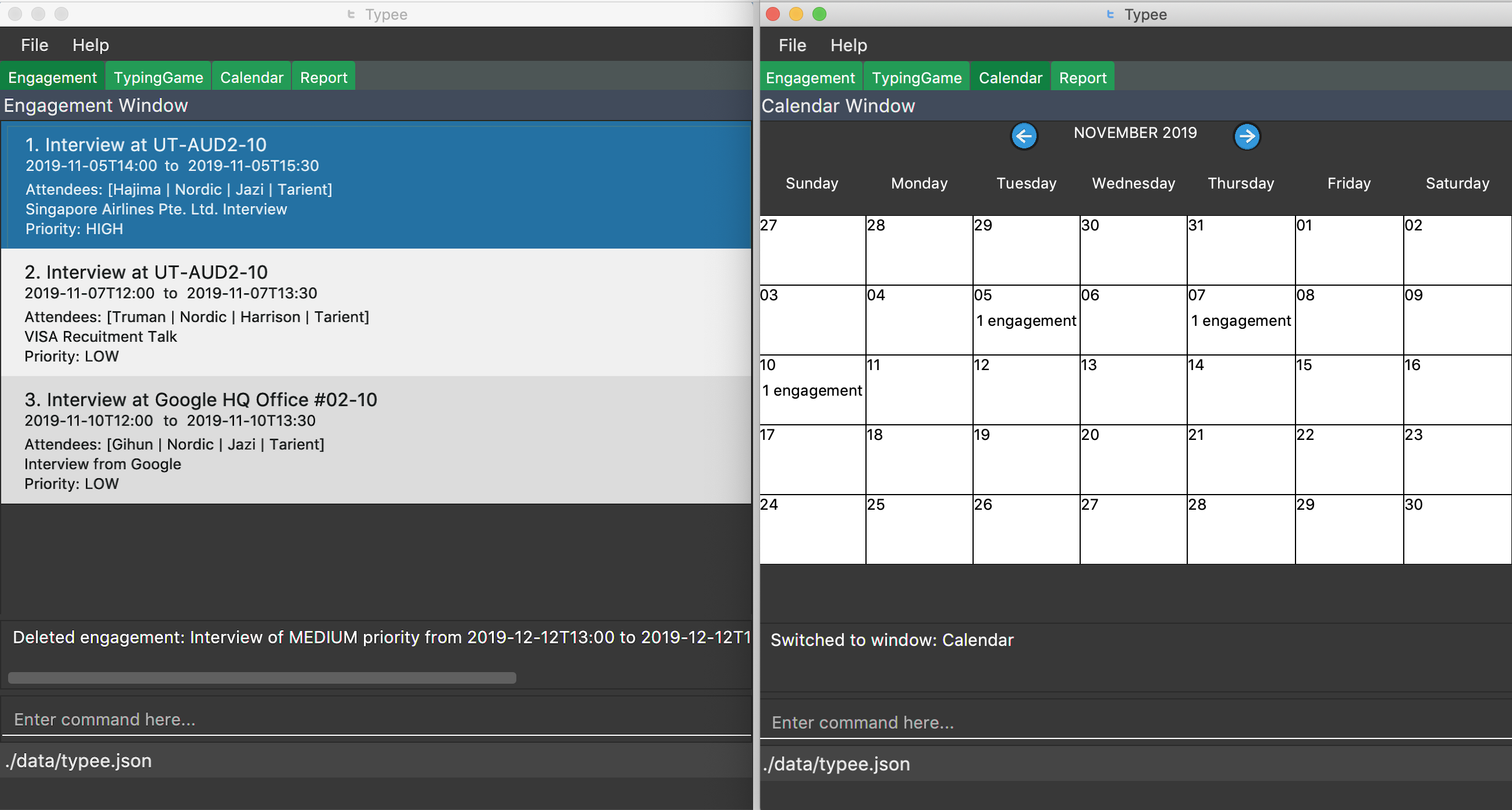
My role was to enhance functionality by designing and implementing new features to the program. The following sections illustrate these enhancements in more detail, as well as the relevant documentation I have added to the user and developer guides in relation to these enhancements.
Note the following symbols and formatting used in this document:
This symbol indicates useful information for users to utilise Typee. |
This symbol indicates additional or important information. |
undo
: A grey highlight (called a mark-up) indicates that this is a command that can be inputted into the command line and executed by the application.
Summary of contributions
Given below is a brief summary of my contribution to the project. |
Major enhancement:
-
Added the ability to undo/redo previous commands
-
What it does: allows the user to undo all previous commands one at a time. Preceding undo commands can be reversed by using the redo command. The undo/redo feature supports operations that modify the engagement list, and provides instant feedback on the graphical interface.
-
Justification: This feature improves the product significantly because a user can make mistakes in commands and the app should provide a convenient way to rectify them. Especially for secretaries whose nature of work requires high productivity over certain periods of time, undo/redo features are efficient ways to revert and start over.
-
Highlights: This enhancement affects existing commands and commands to be added in future. It required an in-depth analysis of design alternatives. The implementation too was challenging as it required changes to existing commands.
-
-
Added the ability to sort engagements by customisable order
-
What it does: the sort feature takes in a parameter of engagements to sort by (e.g. starting time, priority, name etc.) and an ordering method (ascending and descending), and sorts the displayed engagement list accordingly. Operations that require an index of an arrangement can be specified based on the order of such sorted lists. Moreover, the ordering is preserved when operations that modify the engagement list (e.g. adding a new engagement) take place.
-
Justification: This feature is of significant value in actual scenarios, as secretaries usually deal with a massive amount of engagements, ranging from urgent to long-term, and important to less important. Sorting is thus needed in displaying all entries in a customisable order, such that all engagements can be managed in an organised manner.
-
Highlights: This enhancement requires a large number of customised comparators, which is managed using an Enum class. It also affects existing features that depend on the order of the displayed list or modify the list, which requires a good design of implementation and careful tweaks to existing structures.
-
-
Minor enhancement: refactored code from [Address Book - Level 3] to fit the context of Typee
-
Code contributed: [Functional code][Test code]
-
Other contributions:
-
Project management:
-
Managed milestones
v1.2
-v1.4
on GitHub
-
-
Enhancements to existing features:
-
Documentation:
-
Did cosmetic tweaks to existing contents of the User Guide: #112
-
-
Community:
-
Tools:
-
Used graphviz and PlantUML plugins to aid in editing
-
Added Codacy code review to repository (Pull request #197)
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Sorting all engagements : sort
Sorts the list of all engagements in the engagement list by the order specified by user.
Format: sort p/PROPERTY o/ORDER
Properties supported: start (Start time), end (End time), priority and description Orders supported: ascending and descending .
|
Examples:
-
sort
p/start
o/ascending
Sorts the list displayed in ascending order of start time.
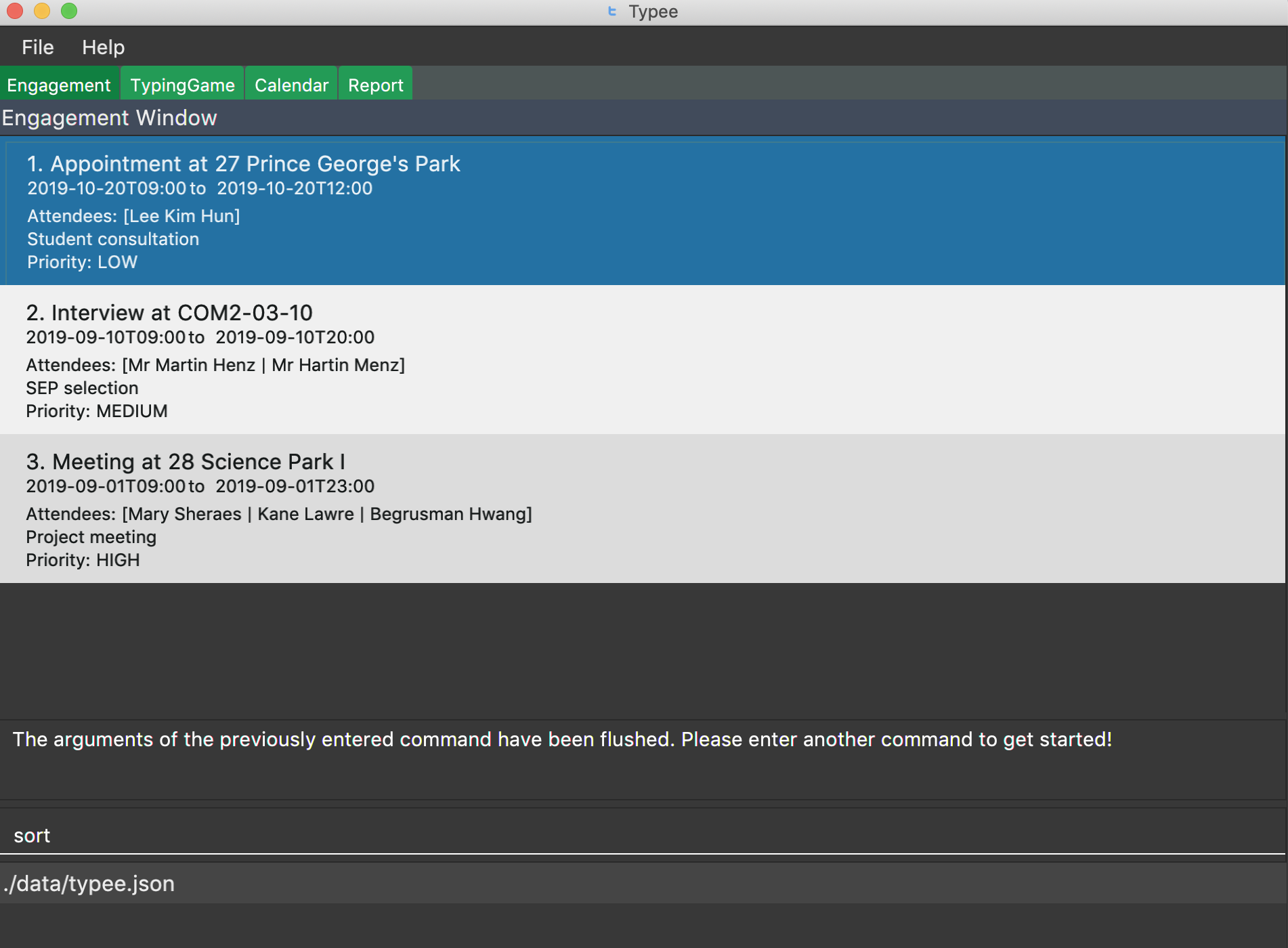
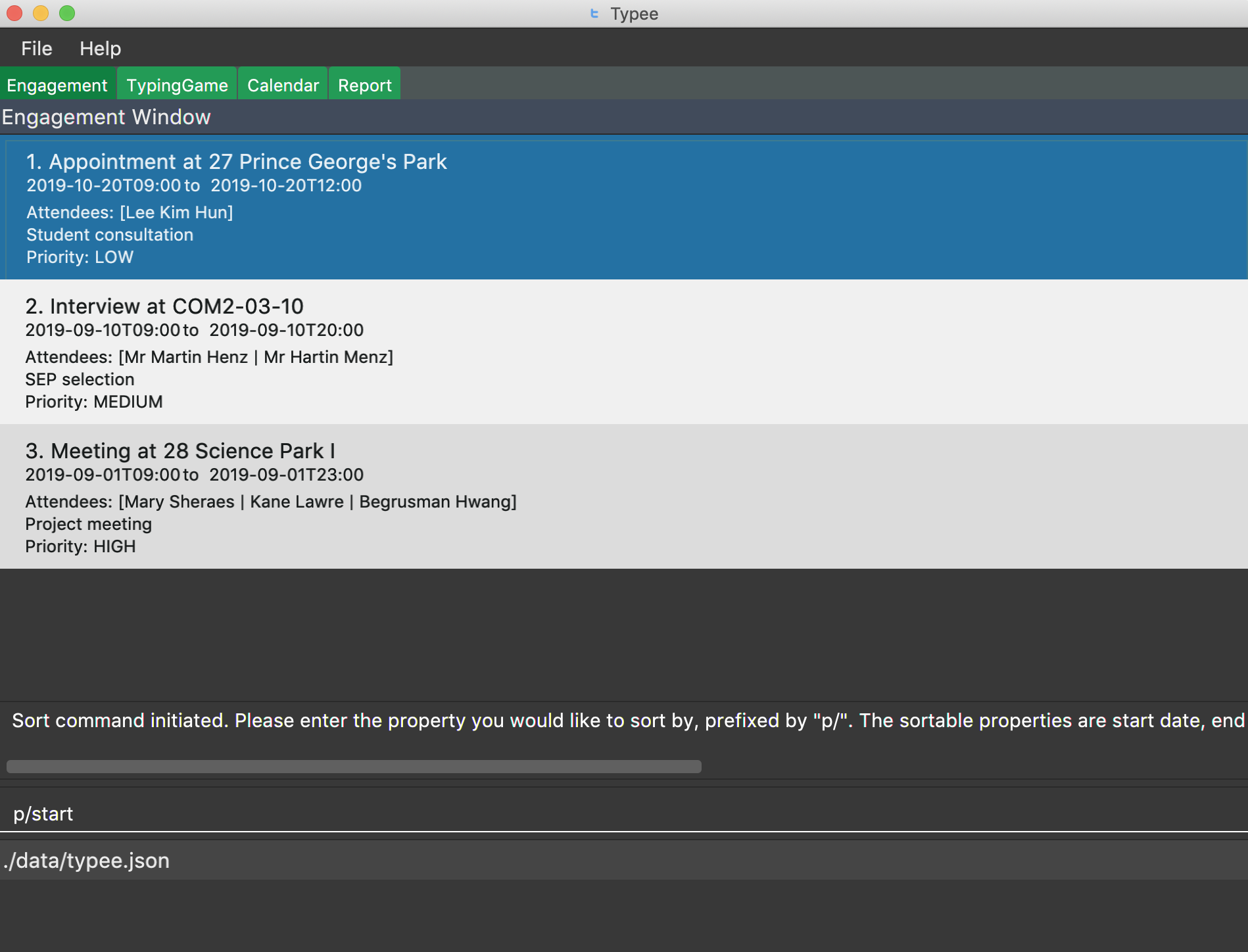
sort
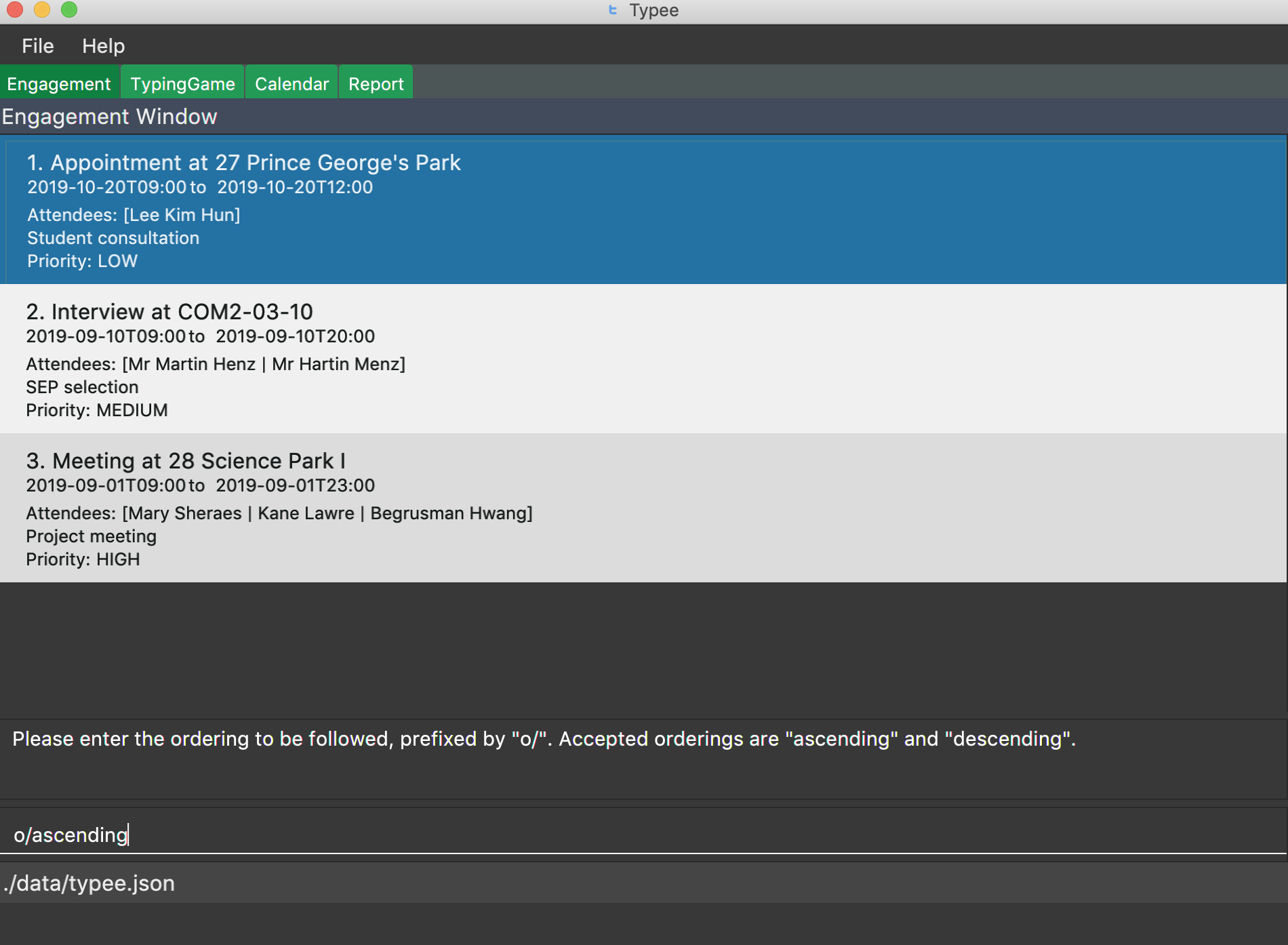
p/start
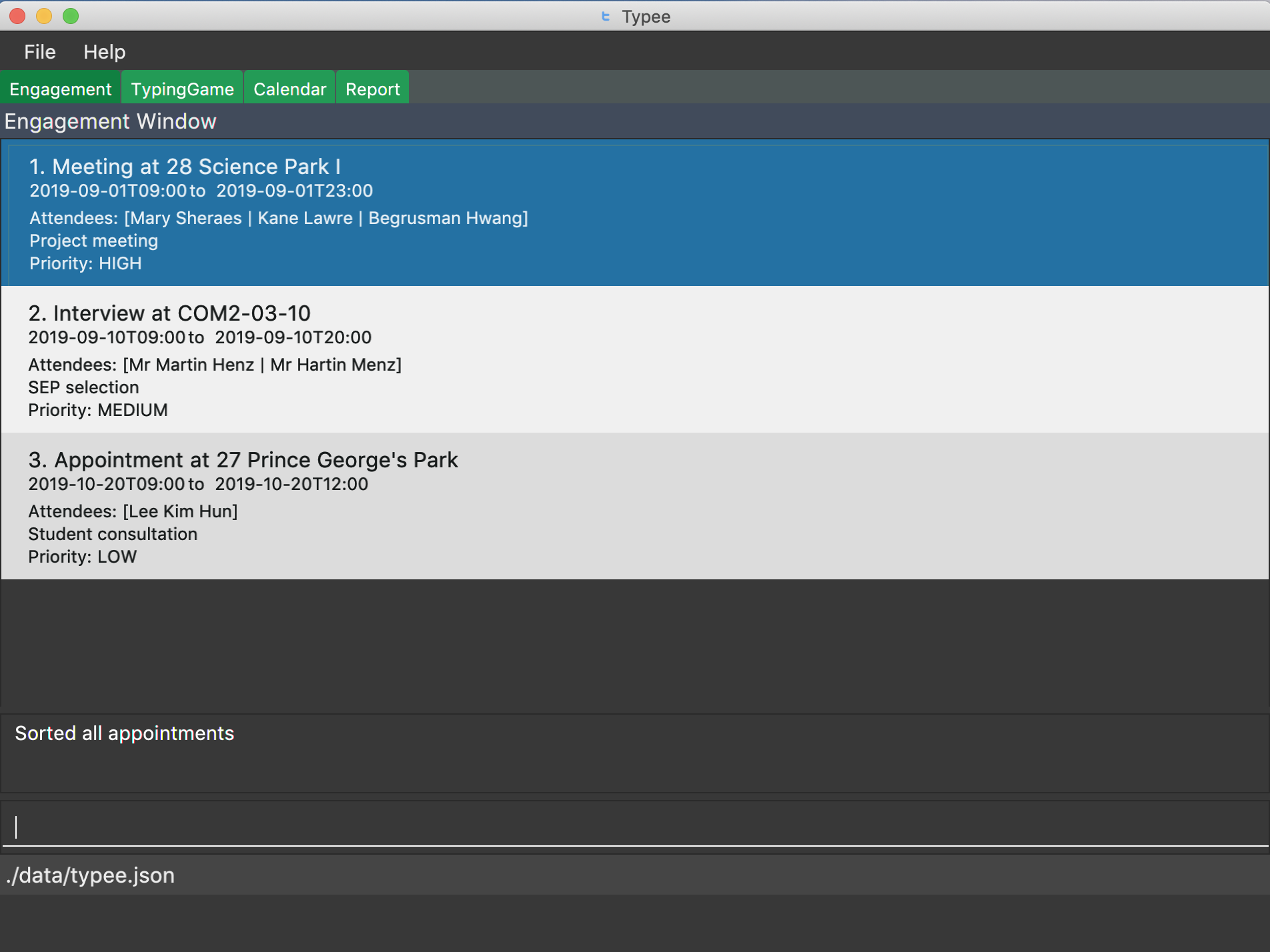
o/ascending
sort command can be done in one shot by giving all attributes at once. e.g. figure below shows the execution of sort p/start o/ascending .
|
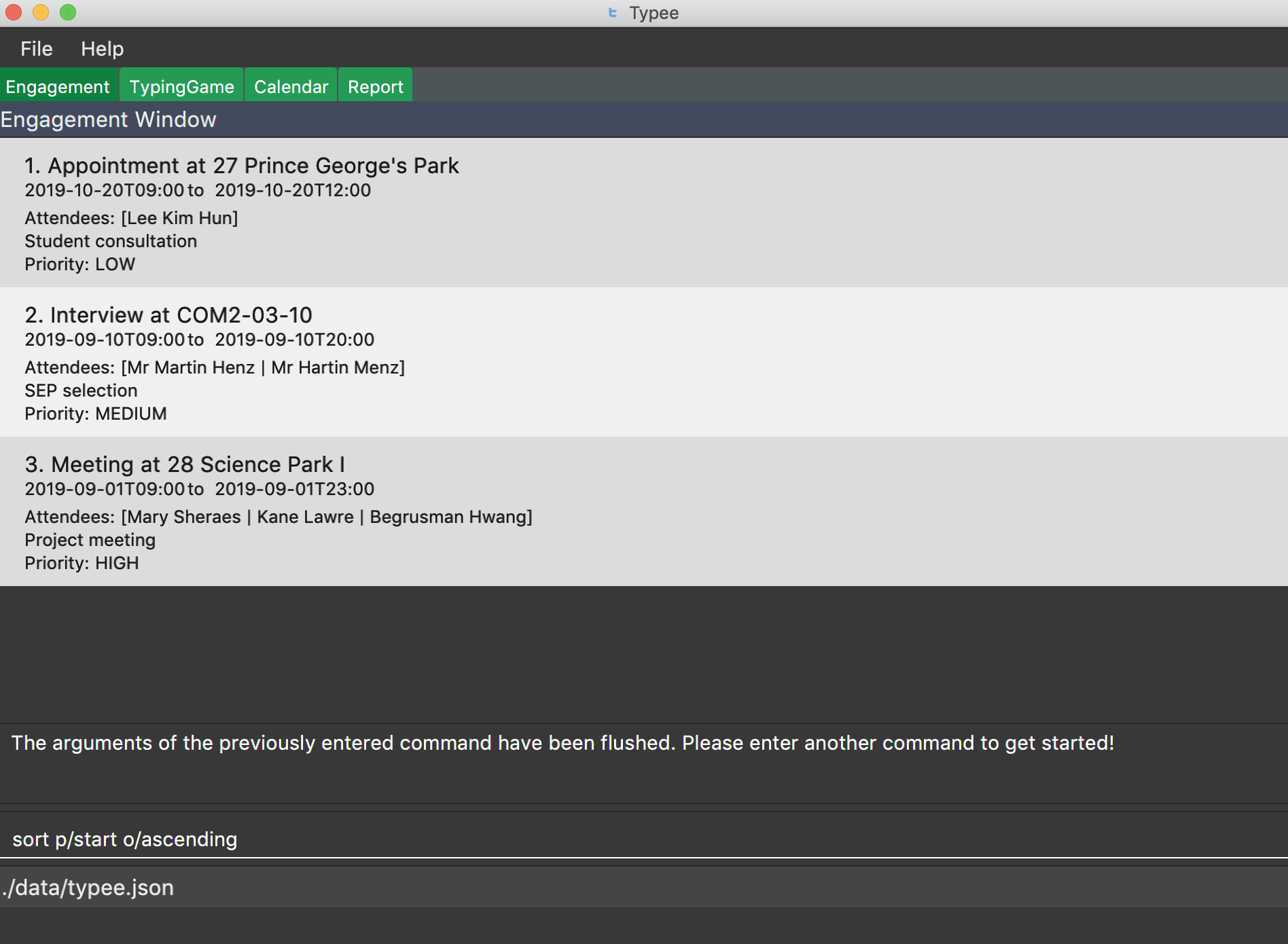
After sorting, commands that require INDEX as an input field (e.g. delete ) will take in INDEX with regards to the new list displayed. e.g. delete i/1 will delete the current first engagement displayed.
|
Undoing the previous command : undo
Undoes the previous command, provided that it exists.
Format: undo
Undo supports the following commands that modify the engagement list: add , delete and clear .
|
Examples:
-
add t/Meeting s/18/10/2019/1500 e/18/10/2019/1800 l/COM-2-B1-03 a/John, Elijah, Sam d/CS2103T Discussion p/High
undo
Undoes the add command, i.e. the engagement with the descriptionCS2103T Discussion
will no longer be in the appointment manager. -
delete i/1
undo
Undoes the delete command, i.e. restores the first engagement in the original displayed list.
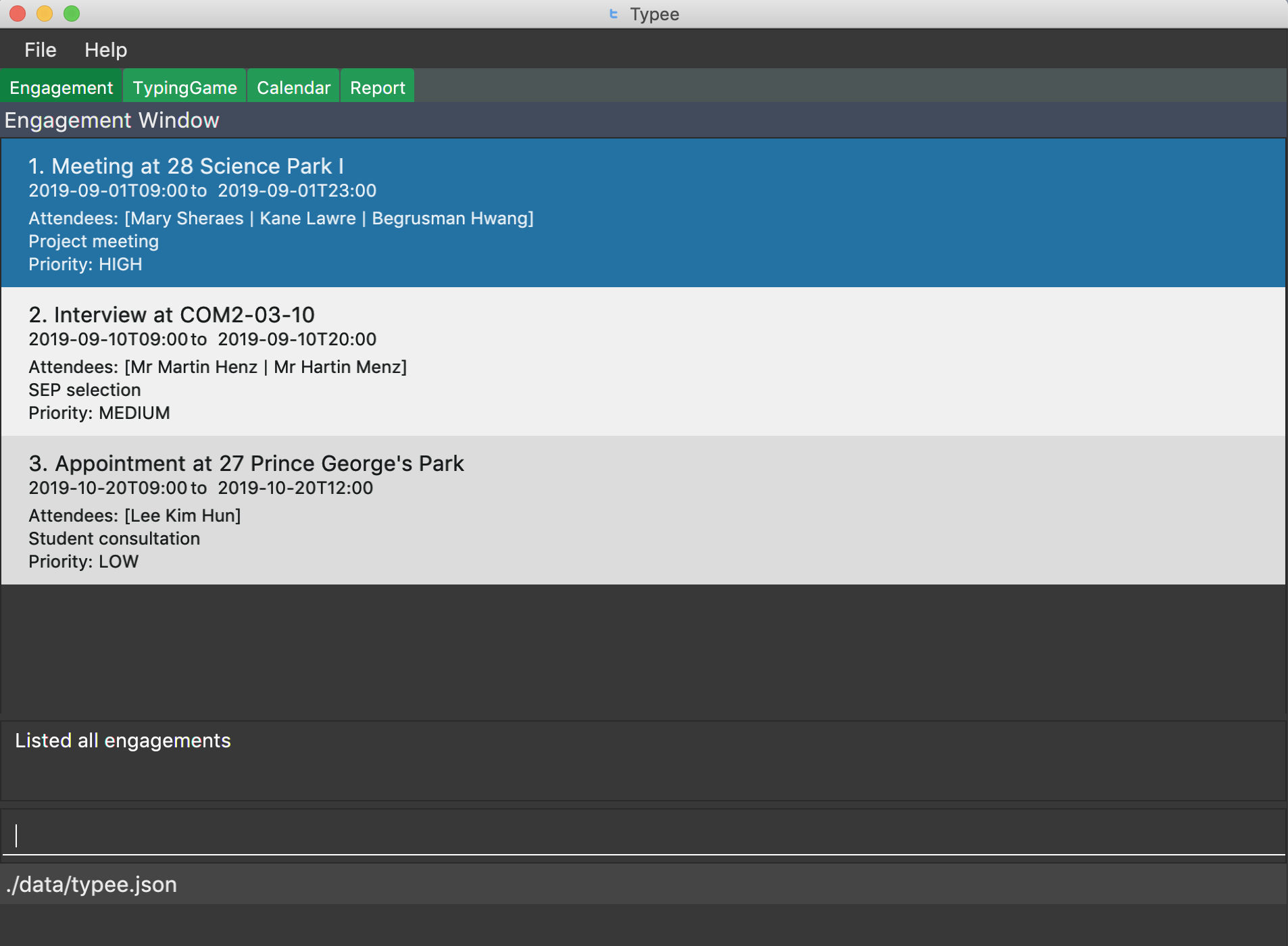
delete i/1
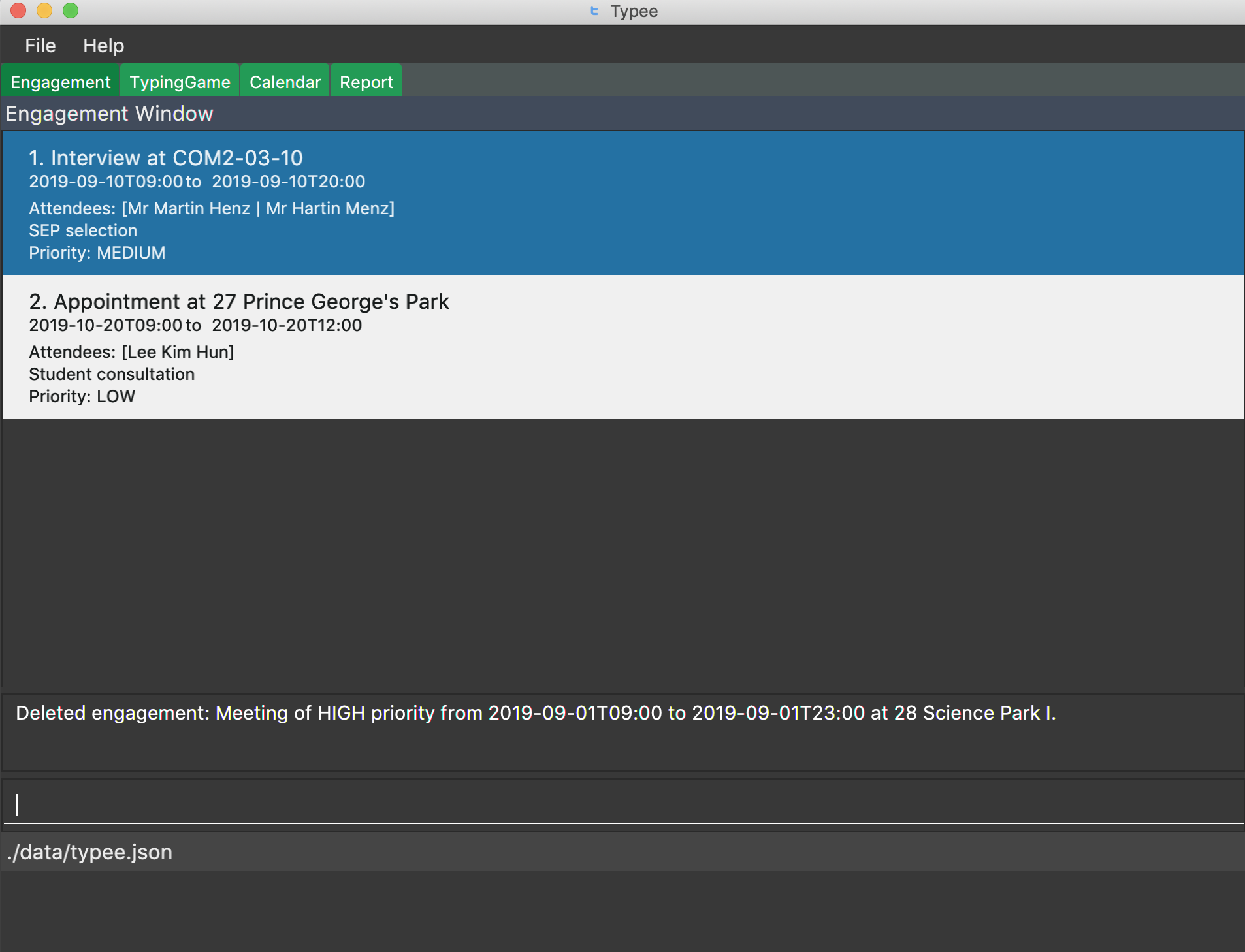
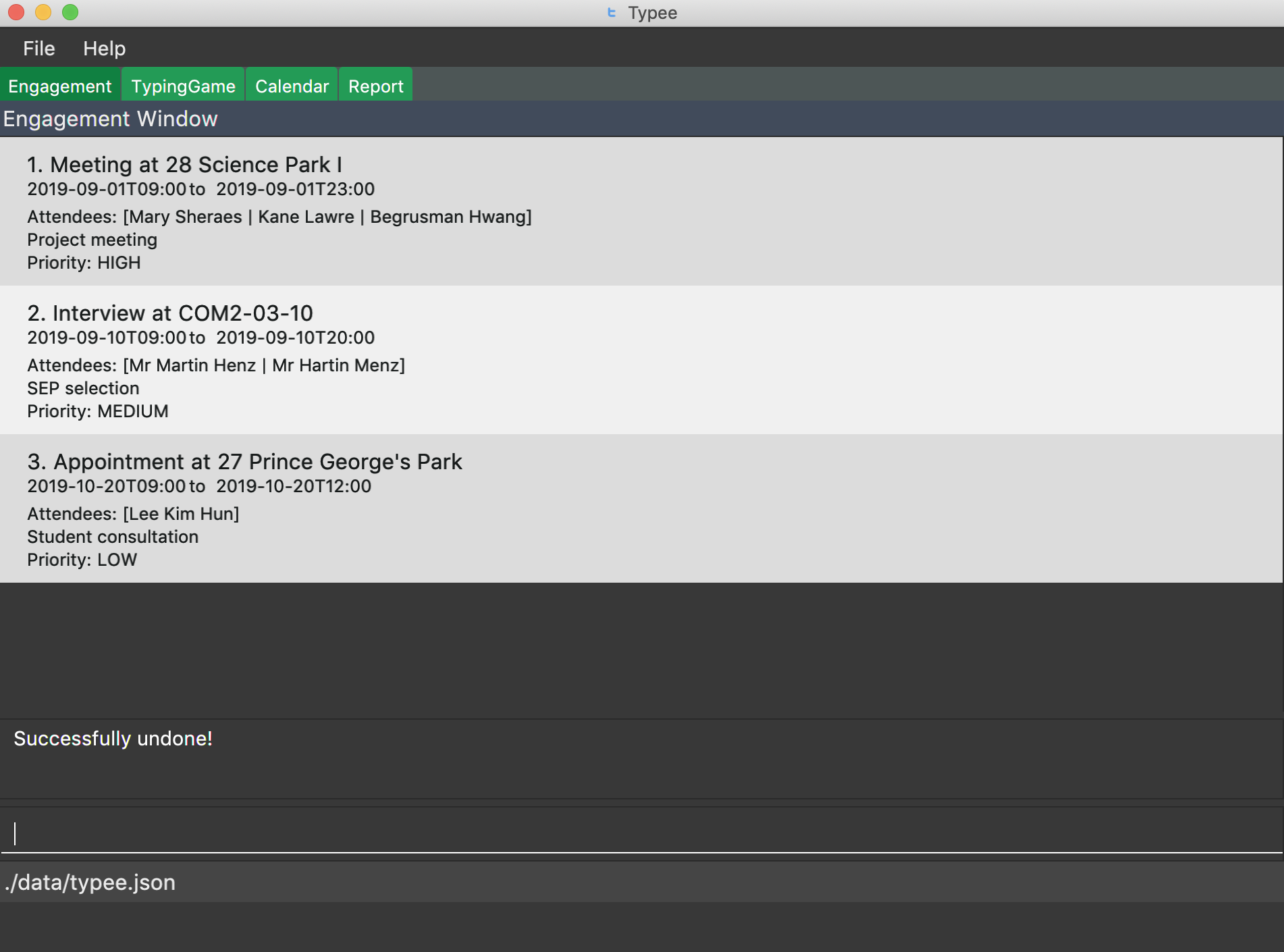
undo
Redoing the previous command : redo
Redoes the previous undo
command. There must be a valid undo command to redo, otherwise this command does nothing.
Format: redo
Examples:
-
delete i/1
undo
(reverts thedelete i/1
command)
redo
(reapplies thedelete i/1
command)
Redoes the previous undo command, i.e. the engagement deleted before undo
will be removed again from the engagement manager.
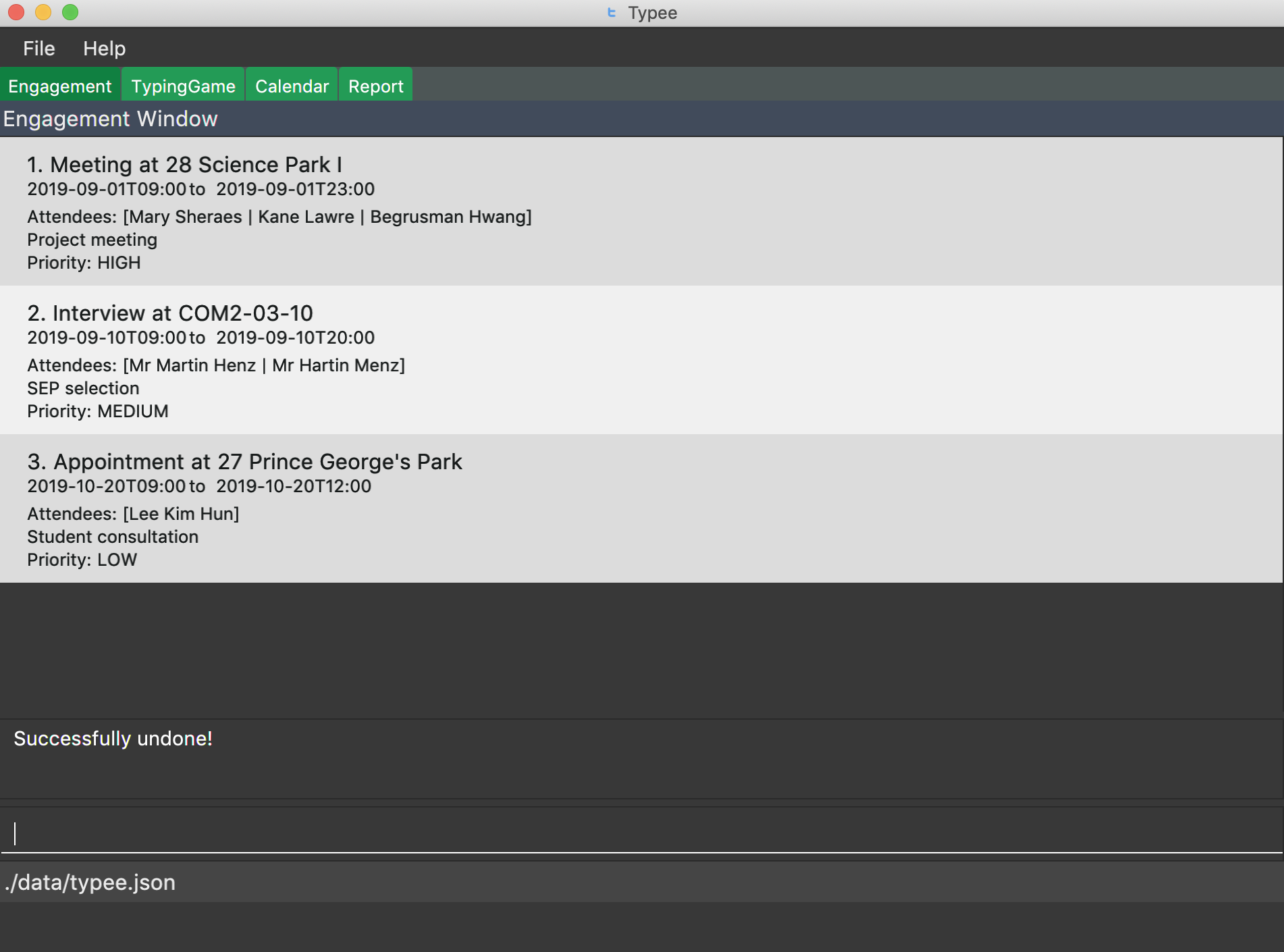
undo
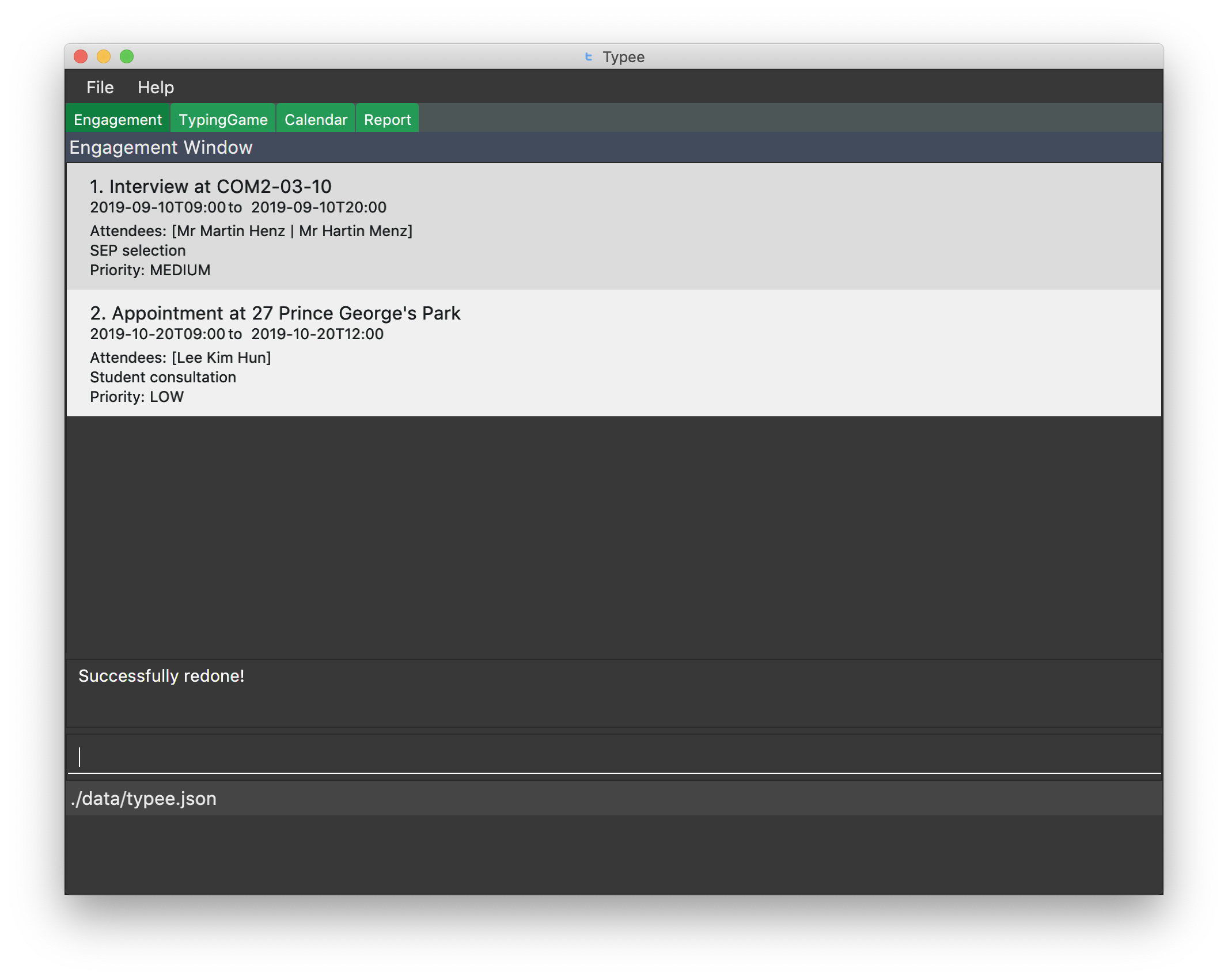
redo
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Sort feature
Implementation
The sort mechanism is facilitated by EngagementComparator
.
The EngagementComparator
is an enum class that implements Java Comparator<Engagement> and specifies the comparing logic of 8 different orders, namely START_TIME
, START_TIME_REVERSE
, END_TIME
, END_TIME_REVERSE
, ALPHABETICAL
, ALPHABETICAL_REVERSE
, PRIORITY
, and PRIORITY_REVERSE
.
Each positive sequence comparator compares two Engagements
with the field specified within its name in ascending order, and _REVERSE
comparators compare in descending order.
Additionally, the Model
interface is modified to support the following methods:
-
Model#setComparator(Comparator<Engagement>)
— Sets the designated comparator in model. -
Model#updateSortedEngagementList()
— Executes the sorting method with thecurrentComparator
in model to sort the internal list. -
Model#getSortedEngagementList()
— Returns the current internal engagement list as an unmodifiableObservableList
.
These operations are implemented in the ModelManager
class as ModelManager#setComparator(Comparator<Engagement>)
, ModelManager#updateSortedEngagementList()
and ModelManager#getSortedEngagementList()
respectively.
Given below is an example usage scenario and how the sort mechanism behaves at each step.
Step 1. The user launches the application for the first time. The currentComparator
will be initialized as null
.
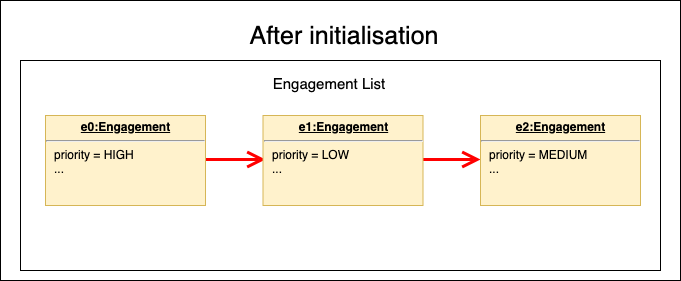
Step 2. The user executes sort p/priority o/ascending
command to sort the engagement list in ascending order of priority. The sort
command calls Model#setComparator()
, causing the currentComparator
in ModelManager to assume the value EngagementComparator#PRIORITY
. The command then calls Model#updateSortedEngagementList
to execute the sorting.
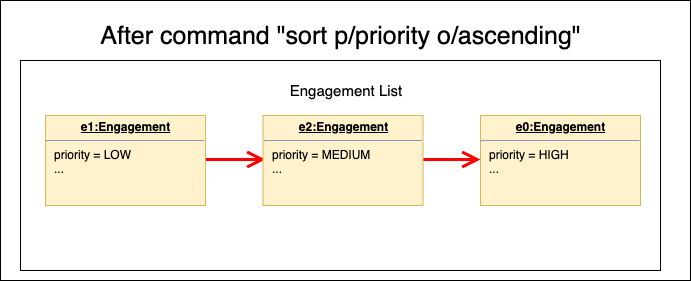
The parsing of sort follows the interactive parsing structure Typee adopts, where various stages are created while parsing. See Sequence Diagram below.
|
The following sequence diagram shows how the sort operation works:
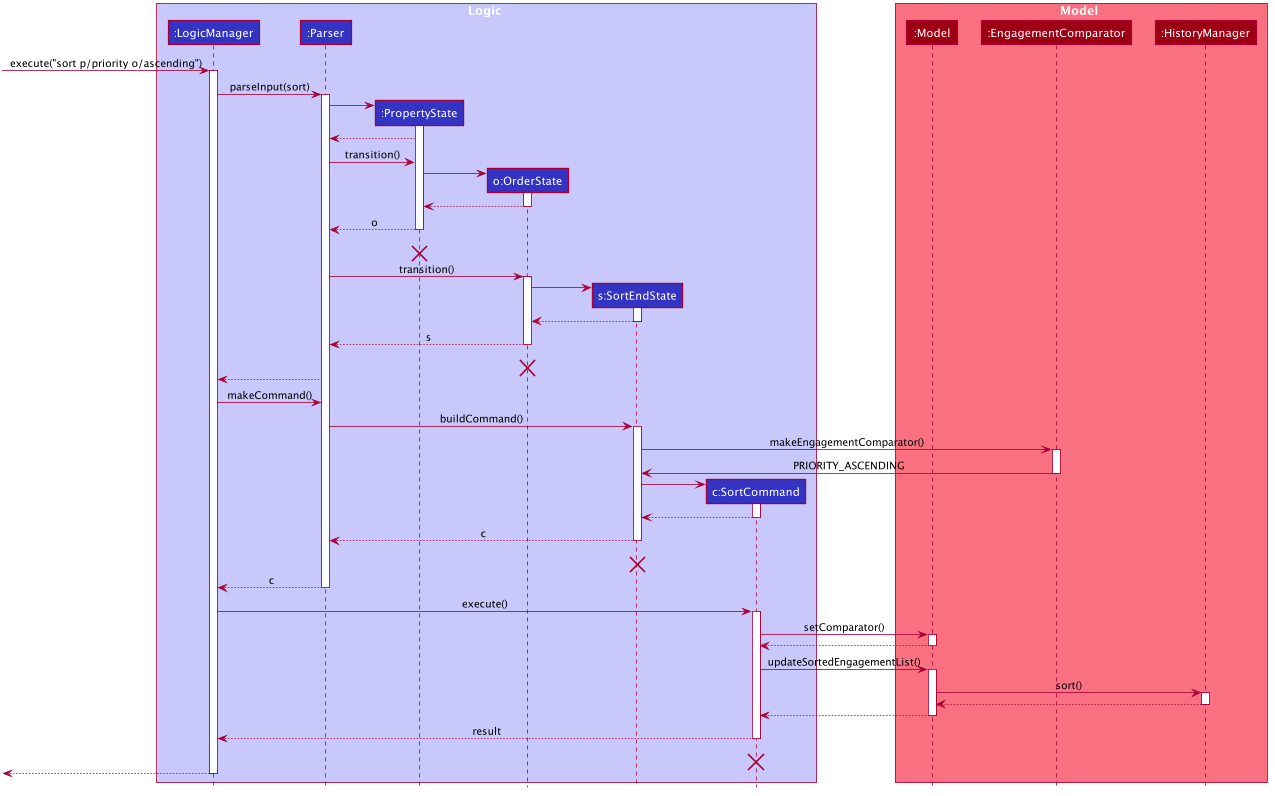
The lifeline for SortCommand and the 3 Stages should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
|
Step 3. The user executes add t/meeting … p/high
to add a new engagement. The add
command also calls Model#updateSortedEngagementList()
with currentComparator
, causing the execution of sorting after the new engagement is added to the list, to preserve the current ordering.
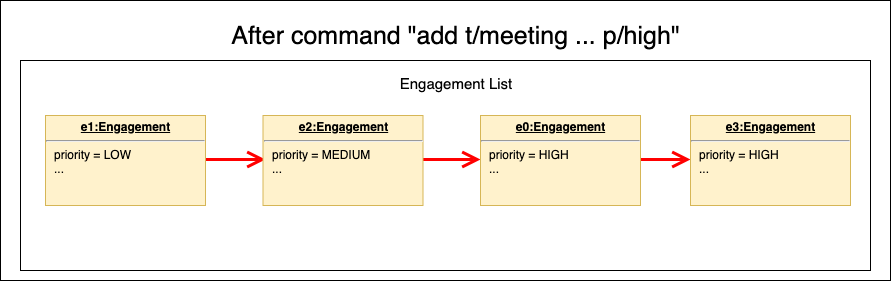
If the currentComparator assumes the initial value null when Model#updateSortedEngagementList is called, the method will simply catch the NullPointerException thrown by java.object.requireNonNull which will essentially abort the attempt to sort with an empty catch block. The initial chronological order is preserved in this case.
|
Step 5. The user then decides to execute the command list
. Commands that do not modify the engagement list or alter the order of the list, such as list
, will usually not call Model#setComparator(Comparator<Engagement>)
, or Model#updateSortedEngagementList()
. Thus, the internal ObservableList
remains unchanged.
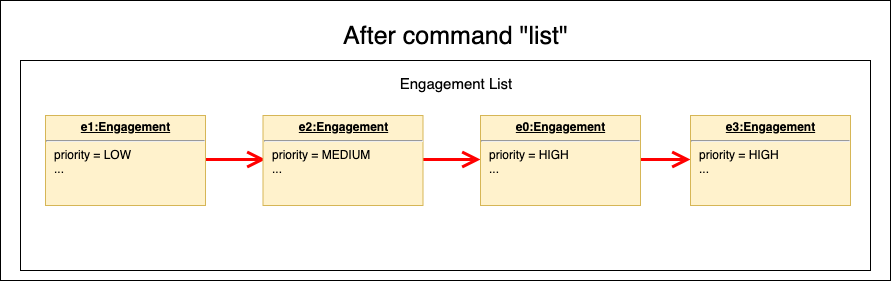
The following activity diagram summarizes what happens when a user executes a new command:
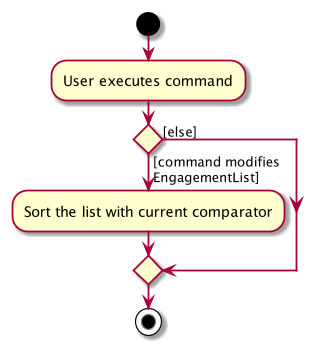
Design Considerations
Aspect: How sort executes
-
Alternative 1 (current choice): Use the
List.sort(Comparator<T>)
function to sort the list.-
Pros: Has trivial support for features that updates the predicate of
FilteredList
, likeFind
. -
Cons: There is a need to sort the list each time a command that modifies the elements of the list is executed, which may result in performance issues in case the list size is large.
-
-
Alternative 2: Replace the
FilteredList
inModelManager
with aSortedList
.-
Pros: Sorting the list is more intuitive, and the ordering of the engagements is automatically preserved whenever a command that modifies the list elements is executed.
-
Cons: Features like
find
command may lose functionality and needs extra modification.
-
Use cases
Use case: (UC09) Undo previous command
MSS
-
User requests to undo command
-
System reverts the appointment list to its previous state
Use case ends.
Extensions
-
2a. There is no previous command
Use case ends.
Use case: (UC10) Redo previous command
MSS
-
User requests to redo command
-
System reverts the appointment list to its previous undone state
Use case ends.
Extensions
-
2a. There is no previous undone command
Use case ends.
Appendix A: Glossary
- Mainstream OS
-
Windows, Linux, Unix, OS-X
- Secretary
-
A person employed by an individual or in an office to assist with correspondence, make appointments, and carry out administrative tasks.
- Manager
-
The person that the secretary is assigned to work for.
- Engagement
-
An arrangement, meeting or interview managed and maintained by a secretary, for the manager to meet someone at a particular time and place.
Instructions for Manual Testing
Sorting the engagement list
(i) Test case:
The sequence of commands is as follows:
sort
p/start
o/ascending
Expected: The list should be sorted in ascending order of start time.
(ii) Test case: Executing the sort command by giving all attributes at once
sort p/start o/ascending
Expected: This should give the exact same result as the previous stepwise command.
Undo and Redo
(i) Test case: Executing the undo command
The sequence of commands is as follows:
delete i/1 (provided there is at least 1 engagement in the list)
undo
Expected: The previously deleted engagement should reappear in the list.
(ii) Test case: Executing the redo command
The sequence of commands is as follows:
delete i/1 (provided there is at least 1 engagement in the list)
undo
redo
Expected: The recovered engagement should disappear in the list.